React is one of the most popular JavaScript libraries used for building user interfaces. With React, developers can create reusable components that can be used throughout an application. React Hooks were introduced in version 16.8 to provide a way to reuse stateful logic between components. A custom hook is a function that uses one or more of the built-in hooks provided by React to create a reusable piece of stateful logic. In this article, we’ll discuss how to create a custom React hook for better code reusability.
Step 1: Identify the Reusable Logic
The first step in creating a custom React hook is to identify the reusable logic that you want to extract into a hook. For example, if you find that you’re writing the same code to fetch data from an API in multiple components, you can create a custom hook to handle this logic in a more modular way.
Step 2: Create the Hook
To create a custom React hook, you need to follow a few conventions. The name of your custom hook must start with the word “use” to ensure that React recognizes it as a hook. You’ll also need to import any built-in hooks that you want to use, such as useState or useEffect.
Here’s an example of a custom hook that fetches data from an API:
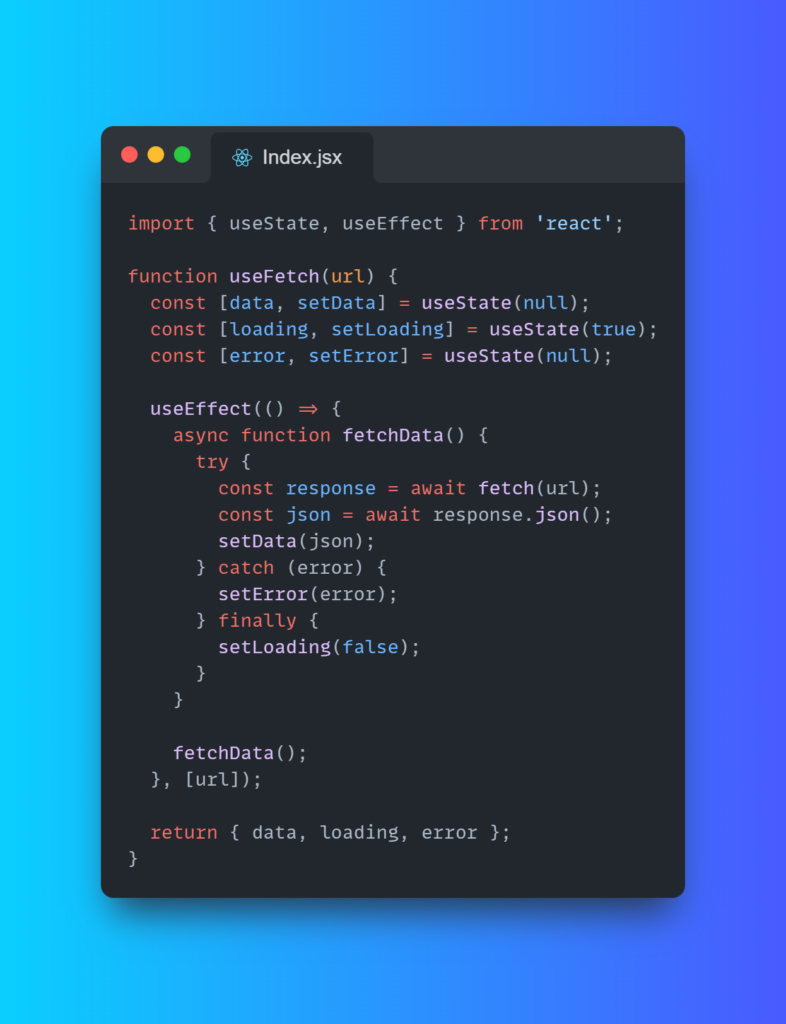
In this example, we use the useState and useEffect hooks to manage the state of our data, loading, and error variables. The useEffect hook is used to fetch data from the API and update the state accordingly. Finally, we return an object with the data, loading, and error values to use in our components.
Step 3: Use the Hook in Your Components
Once you’ve created your custom hook, you can use it in your components just like any other hook. Here’s an example of how you can use our useFetch hook in a component:
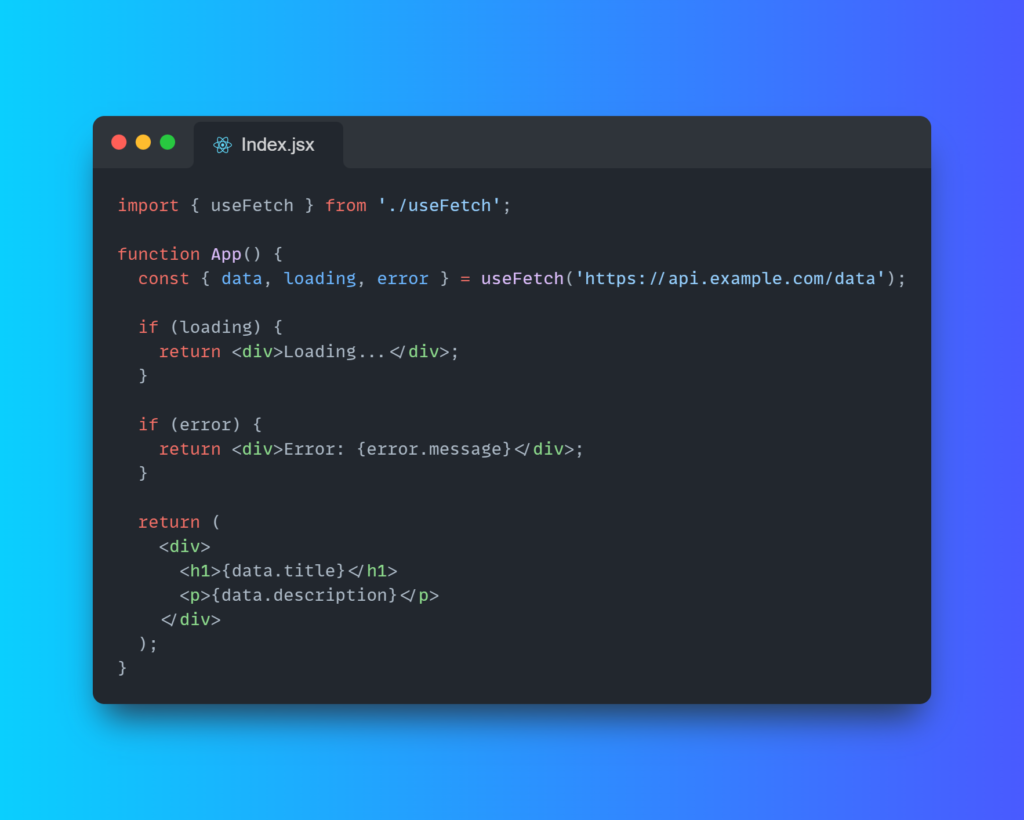
In this example, we import our useFetch hook and use it to fetch data from the API. We then render the data in our component using conditional statements to handle loading and error states.
In conclusion, creating custom hooks in React is a powerful way to extract reusable logic from your components and make your code more modular and reusable. By identifying reusable logic, creating the hook, and using it in your components, you can make your code more efficient, easier to read, and easier to maintain. With version 18, React continues to provide developers with more ways to create custom hooks and improve the reusability of their code.