React is a popular front-end library for building user interfaces. One of the reasons for its popularity is its flexible and powerful component system. In this article, we will explore two advanced React patterns: Higher-Order Components (HOCs) and Render Props. These patterns enable us to reuse code, abstract functionality and create more flexible and composable components. We will dive into how to use HOCs and Render Props in React.
Higher-Order Components (HOCs):
Higher-Order Components (HOCs) are functions that take a component and return a new component. The returned component can have additional functionality or props that the original component did not have. HOCs are useful for code reuse and abstraction of functionality. Let’s look at an example of how to create a HOC in React.
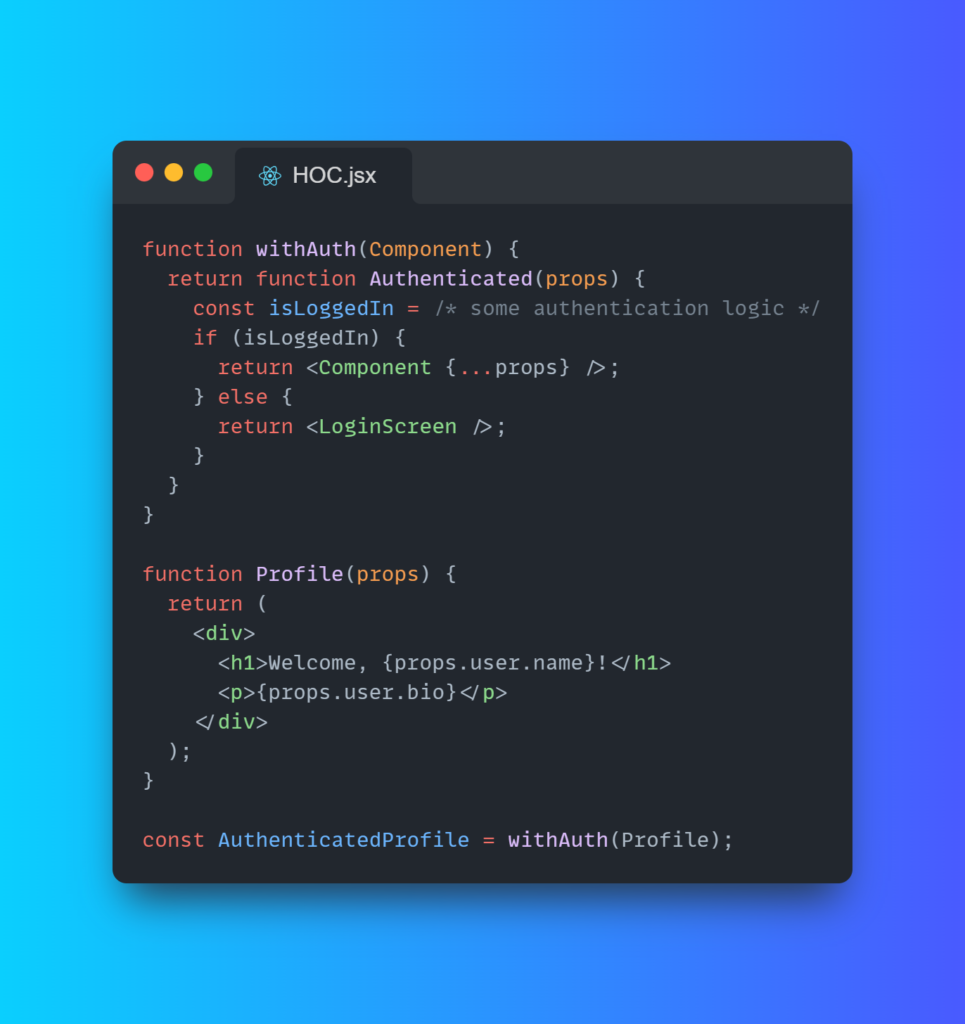
In this example, we created a HOC called withAuth that takes a component and returns a new component that checks if the user is authenticated. If the user is authenticated, it renders the original component (Profile), passing all the props down to it. If the user is not authenticated, it renders a LoginScreen. We then use withAuth to create a new component called AuthenticatedProfile, which is our original Profile component with authentication logic added to it.
Render Props:
Render Props is another advanced React pattern that allows us to pass a function as a prop to a component. The function returns an element that the component can render. This pattern is similar to HOCs in that it enables code reuse and abstraction of functionality. Let’s look at an example of how to use Render Props in React.
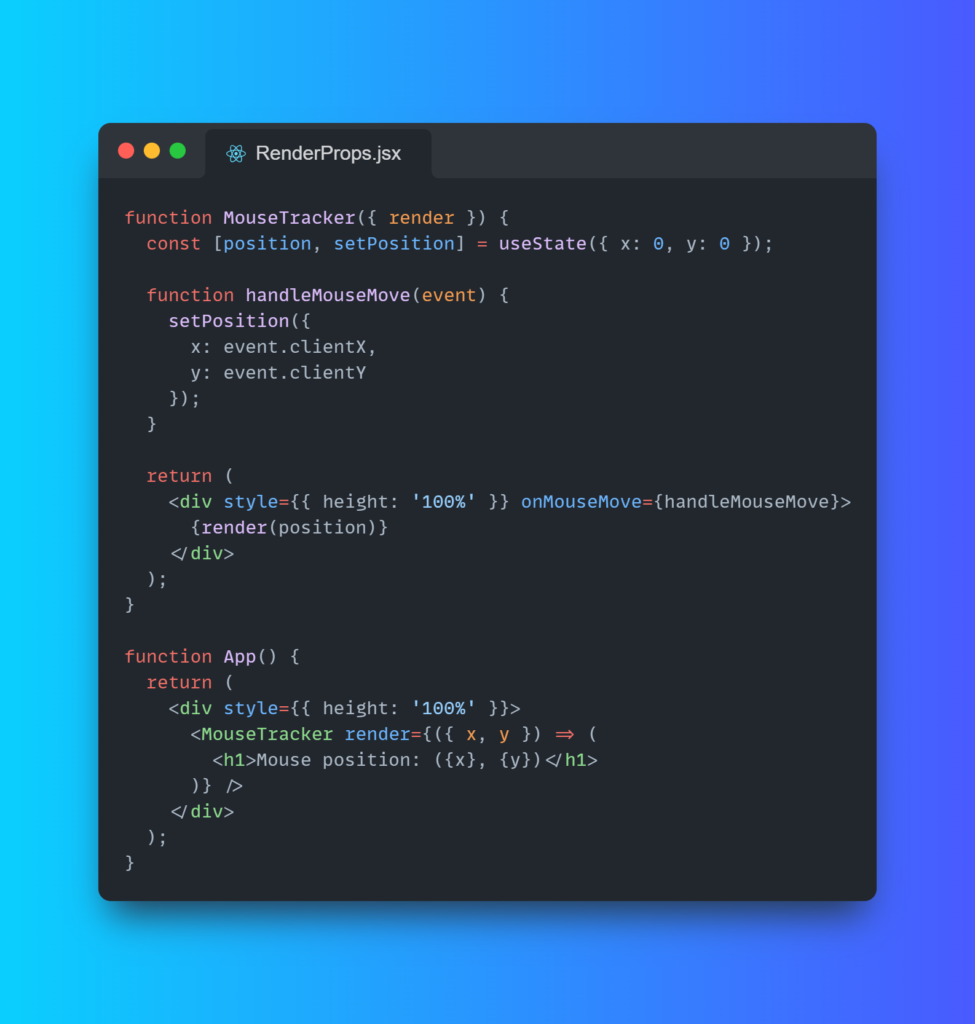
In this example, we created a MouseTracker component that tracks the mouse position and renders the result of a function that we pass as a prop. The function takes the mouse position as an argument and returns an element to render. We then use MouseTracker in our App component, passing a function as a prop that renders an h1 element with the mouse position.
Conclusion
In conclusion, Higher-Order Components (HOCs) and Render Props are powerful React patterns that enable us to reuse code, abstract functionality, and create more flexible and composable components. HOCs are functions that take a component and return a new component with additional functionality or props. Render Props is a pattern that allows us to pass a function as a prop to a component, which returns an element. Both patterns can be implemented with functional components, making it easier to write and maintain complex applications in React. By using HOCs and Render Props, we can create more modular and reusable code, which is crucial for building scalable applications. These patterns are powerful tools that can help us write better, more maintainable code, and they are essential to master for any serious React developer. With this, we can continue to use these patterns to create high-quality, performant applications.